Coding is like building with blocks – you need the right pieces to create something amazing and unique. In this post, I will be showing you the most basic programming elements that will be the building blocks of your programs. I will give you examples and tips on how to properly integrate and utilise these elements in your code so that you can start writing your own code in no time!
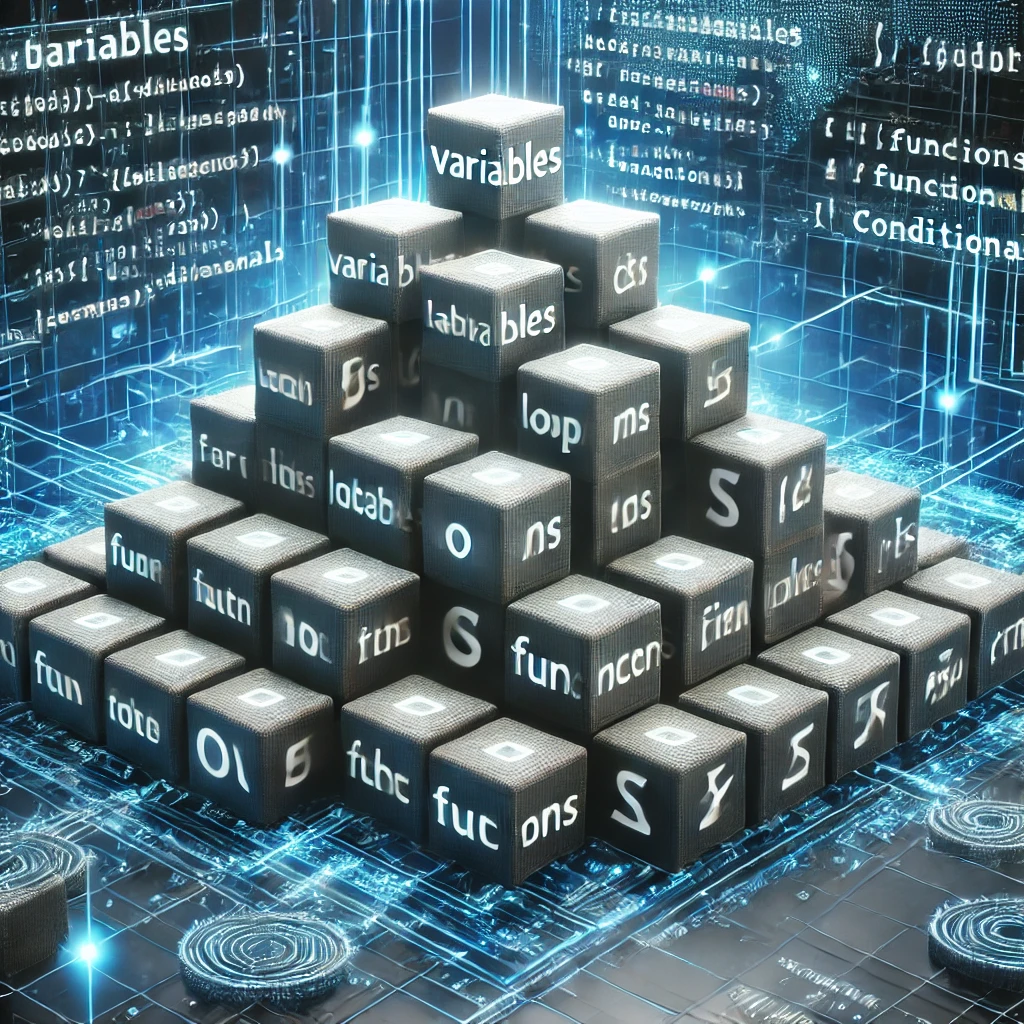
Variables: Storing Information
Variables are like boxes that hold information. Think of it like a locker at school: you can label the locker with a name and store things inside it, like books, gym clothes or food. Similarly, in coding, variables hold numbers, words, or other data that your program needs to remember. You can name the box and put something inside it, like a number or a word. They’re essential because they let programs remember things while they’re running.
age = 25
This is an integer variable, it stores integers (whole numbers) and is useful for doing math.
name = "Alice"
This is a String Variable. These are used to store sentences or phrases that consist of more than 1 character. A character variable is a variable that only stores 1 letter / number but it’s basically an inferior string and can be defined as a string either way.
Balance = 305.50
Above is an example of the float data type. This is basically an integer but it can store decimal points and takes up more memory space as a result of that. Mainly used for money values or fraction values.
passwordCorrect = True
The above is a boolean (bool) variable which stores a true / false, a Yes / No or a 1 / 0. It is used to check if something is true and mainly used in if / else loops or while true loops. If the condition is true, then execute A if false, execute B.
Tips:
When creating variables, choose names for variables that describe what they hold so if a variable is for someone’s bank balance, the name of the variable should be “bbalance” or “bank_balance” to make sure that whenever it is time to call a variable it is easy to find its name. When working with a variable, you may want to change what data value the variable holds after a certain interval passes or a certain condition has been met. You can do this by simply re-defining it as such:
age = age + 1
Using variables makes your code reusable and easier to understand. Instead of hardcoding values (re-typing them every time you want to use them), you can store them in variables and update them later for more efficient usage. When making variable names, don’t use names that programming languages already reserve, like print or list and if you must, change your variables so that they are still distinct from the reserved ones. For Example, “print” could become “Produce” or “User_print”. And for variables like these, use commenting to clarify what they are for.
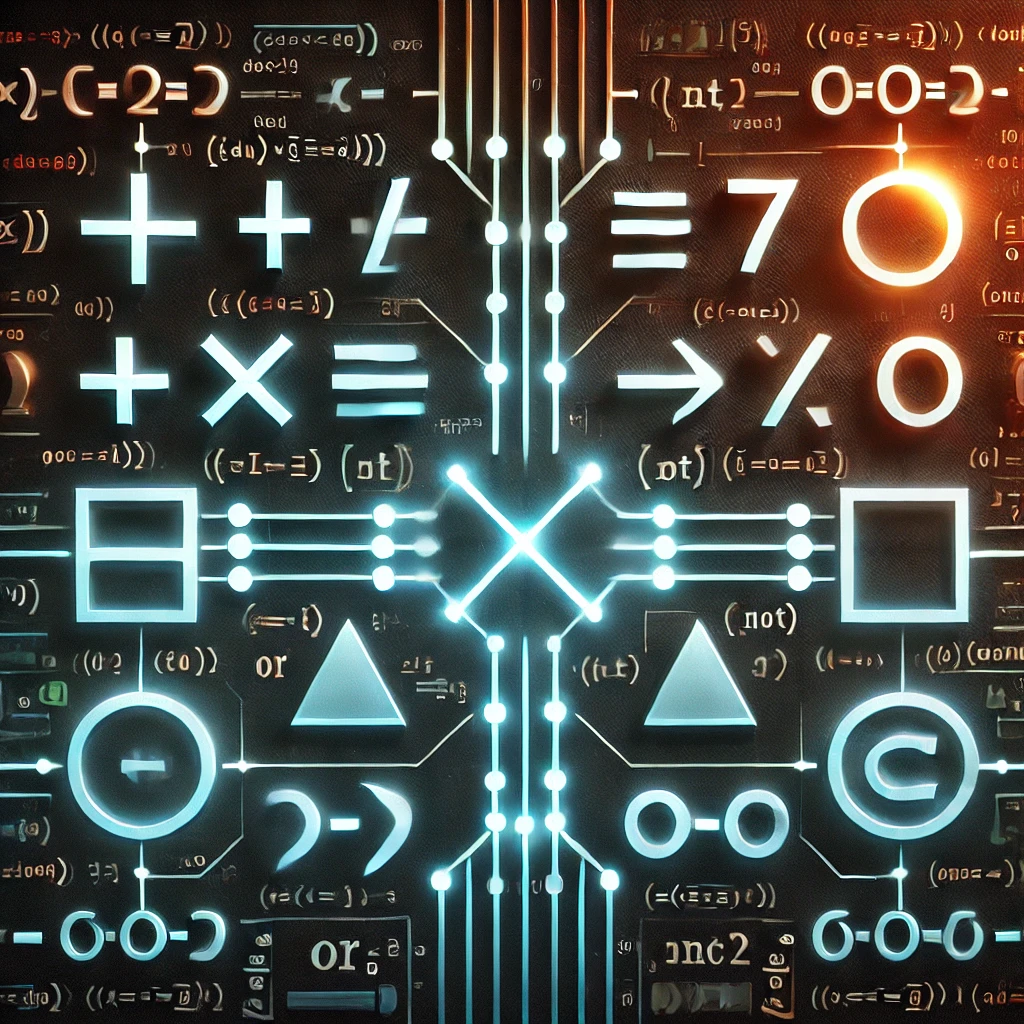
Operators: Doing Math and Comparisons
Operators are symbols like + or > that let you do mathamatical operations and compare data sets. They’re used to perform actions or evaluate conditions in your code.
Types of Operators:
- Math Operators:
+
,-
,*
,/
These are your simple addition, subtraction, multiplication and division operation that are mainly used for basic mathematical calculations while running code.- For Example:
result = 5 + 3
- For Example:
- Comparison Operators:
==
,!=
,<
,>
These compare a variable against a value to check if the statement that it has been told to check is true or not. If it is, the code continues, else it jumps forward or returns an error message.
== means is equal to, != means is not equal to and > and < are pretty well know but mean greater than and less than.- For Example:
is_equal = (5 == 3)
# False
- For Example:
- Logical Operators:
and
,or
,not
These operators allow you to add another level of variability to your code and allow for advanced condition checking like ”if_age < 25 and > 18 then
which helps save lines of code and allows to create unique outcomes to every unique input.“
- For Example:
is_valid = True and False
# False
- For Example:
Tips:
When coding using math operators, remember that some operations happen before others like we were taught in school using BIDMAS / BODMAS / PEMDAS or whatever you were taught in school. Use parentheses to make which operation you want happening first clear:
result = (5 + 3) * 2
When you plan on adding math operators to your code, test operators in small pieces of code or in other small projects to understand how they work so that your main code does not start encountering errors everywhere. Comparison operators used for comparing a variables and its desired output, return True or False based on whether the condition was fulfilled or not whereas logical operators combine multiple conditions to check if they are all correct at the same moment instead of having to do if / else loops in if / else loops to achieve the same outcome.
Functions: Organizing Code
Functions are named blocks of code that you can reuse. They increase your code’s clarity and make the code easier to debug when an error arises.
Example:
def greet(name):
return f"Hello, {name}!"
print(greet("Alice")) # Output: Hello, Alice!
Tips:
When creating functions, keep functions short and focused and only write what you need them to do. Do not overcomplicate functions. After a function has been made, add comments by using “#” to explain what the function does so you don’t forget or have to read the code to remember. For the name of the function, use a descriptive name so others (collaborators you may be working with or even yourself) understand what the function does. Use default values for parameters when possible:
def greet(name="Guest"):
return f"Hello, {name}!"
Functions are very useful because of their versatility and because they let you avoid repeating code and make it easier to update your code later.

Conditional Statements: Making Choices
Conditionals let your program decide what to do based on the situation. They’re used for decision-making based on checks that it makes to values stored in your code.
if bbalance >= 500000:
print("You’re rich!!!")
This is an if statement this checks the provided statement and if the statement to check is true, then it completes the following task and if the statement is not true then it skips the next part and moves on.
age = 20
if age >= 18:
print("Adult")
else:
print("Minor")
This is an if-else statement. Basically an if statement but it also tells the code what to do if the checked condition is false.
if age < 13:
print("Child")
break
elif age < 18:
print("Teenager")
break
else:
print("Adult")
By adding “elif” to the mix, you get the code to handle multiple conditions by checking additional conditions if the initial one is false. This allows for more compact if / else statements which help prevent nested if / else statements where an if statement is inside another just to get the desired output.
message = "Adult" if age >= 18 else "Minor"
print(message)
This is an example of a Ternary operator which is just a more compact way of writing conditional statements again, used to optimise space especially on larger projects. The downside here is you cannot employ the “elif” conditional statement.
Tips:
When using conditional statements, always include an else statement to handle unexpected cases that may arise from checking conditions. After you have created a conditional statement, on a separate test project, test different inputs to see how the conditions behave and make sure they match what you want / were expecting. Remember to use if, elif, and else for decision-making as they help to simplify all decision making processes in your code. Remember to also combine conditions with and / or statements:
if age > 12 and age < 18:
print("Teenager")
Loops: Repeating Tasks
Loops let you do the same task over and over. They’re essential for working with lists or automating repetitive actions that may be occuring during the operation of the code.
- For Loop: A for loop is used when you know how many times you want to repeat an action or have a specified variable that has an integer value that can be read by the code when the loop is run. For example:
for i in range(5):
print(i)
- While Loop: While loops are implemented when you want to repeat an action as long as a condition is true and after the condition becomes false, the while loop ends and the compiler moves on. For example:
x = 0
while x < 5:
print(x)
x += 1
- Do-While Loop (This isn’t available in Python but is very common in other programming languages): These loops ensure that the loops run at least once before checking the condition. For example, in JavaScript:
let x = 0;
do {
console.log(x):
x++;
} while (x < 5);
Each type of loop is unique in its own way in that it helps the code run effectively in its own way, so choose based on what your program needs.
Tips:
When creating loops, always make sure your loop has a way to stop or an end point so that you don’t end up in an infinite loop. When trying to manipulate your loop to create an ideal outcome, use essential and basic code like ”break“ to stop the loop after a condition has been met or “continue“ to skip to the next repetition of the loop. And before implementing them, test loops with small data sets to avoid infinite loops.
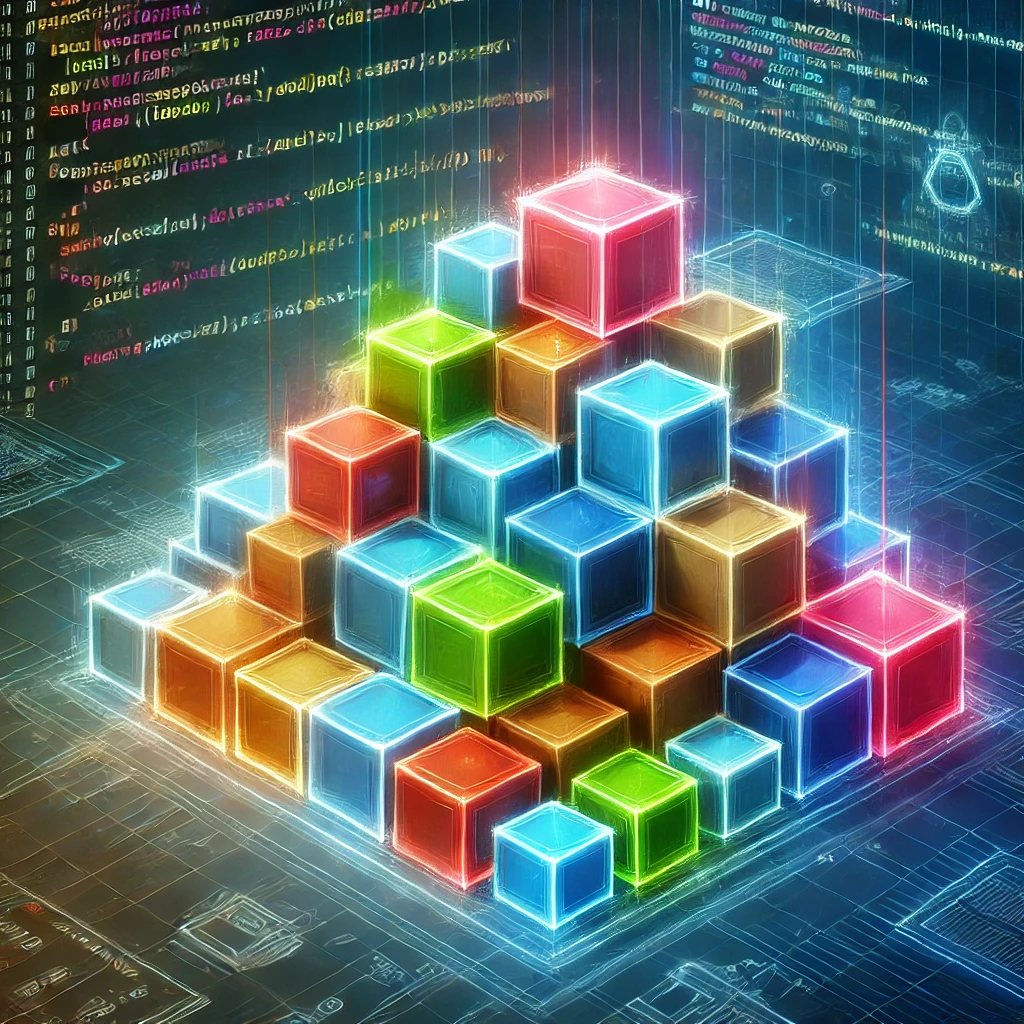
Arrays (Lists): Grouping Data
Arrays (Lists) are collections of items, like a grocery list. They let you store and work with multiple pieces of related data in one place.
Example:
fruits = ["apple", "banana", "cherry"]
This is a classic array and if you learn how to code online then it’s probably one of the few examples of arrays that you will be shown when learning about them. This is a list that is applied to a name that you can call on later to allow for quick access to data that may be needed later on in your code.
fruits.append("date")
This is what to write when you are trying to add data to the array. By calling this function, you are adding “date” to the back of your dictionary after this piece of code is completed, if you printed your array, the array would output “apple, banana, cherry, date” instead of just “apple, banana, cherry”.
print(fruits[1]) # Output: banana
When you add a set of square brackets ( [ ] ) next to the name of the array, and type a number in it, this tells the array that you only want the value that is on that position. When calling from an array, the first data set has the value of 0 instead of 1, the second has a value of 1 instead of 2, so on and so forth so when calling specific data from an array remember to – 1 from the position of data you want to call.
fruits.remove(“banana")
This code does the opposite of what “fruits.append()” does. This removes the specified data value and clears its space for the next time in line. When using this, if you have 2 of the same data value in the array, it will remove the first instance this happens in and if the array doesn’t have the specified value, it will raise an error.
fruits.pop(2)
This has the same functionality as the aforementioned function except instead of typing what you want removed from the array you type the index of the value that you want removed. So if you want to remove the “apple” instance, you would type:
fruits.pop(0)
And this would remove the “apple” value from the array. If there is no index value provided, then this function will simply remove the last value in the array by default. Meaning if you write “fruits.pop()”, it would remove “date” from our example array.
fruits.clear()
Just as the name suggests, the “.clear” function just entirely clears the array so after it has been called, if a print function is called as such:
print(fruits)
The output area will be blank as there is no data left in the array for it to print.
Tips:
Lists are used when you need to store multiple related items together for easier calling and manipulation. When using arrays, be careful not to call for an item or index that doesn’t exist, like fruits[10]. Because this will break your code and just return an error message. To avoid this use if / else checks to make sure the desired value or index exists first before calling them. For a specific value use:
if "cherry" in fruits:
fruits.remove("cherry")
And for index values, check the length of the array using “.len()” before calling on an index value like this:
if len(fruits) = 11
fruits.pop[10]
Then by adding “Else
” statements, you can add what you want the code to do if the “if
” condition is not met. Remember to explore list methods like “.pop()
”, “.sort()
”, and “.reverse()
” to efficiently manipulate the array to help run your code with fewer lines of code and more smoothly and effectively. Another thing to keep in mind is that the first item in a list has index 0 so when you are trying to extract the first item in an array with index calling, always start with 0. When coding, lists can grow or shrink as you add or remove items using “.append()
” or “.remove()
” any of the other methods mentioned before.
Dictionaries: Matching Keys to Values
Dictionaries store data in pairs, like a literal dictionary which you use to find the definition of words. It stores a variable and its value pair. They’re great for organizing data you want to look up quickly.
user = {"name": "Alice", "age": 25}
This is how to define a dictionary. When defining it, make sure the correct format is used otherwise the dictionary will be incorrectly defined and will break when you try to call from it.
user["email"] = "alice@example.com"
This is what to use to add more data to the dictionary that was made before.
print(user["name"]) # Output: Alice
And this is how to call on your dictionary and its stored data.
Tips:
Dictionaries are used when you need to look things up quickly. It’s basically an advanced array. Keep keys short but meaningful so that they are easy to remember and they dont take up too much memory when your program is run. When trying to call multiple values from a dictionary, use loops to process all of the key-value pairs. In dictionaries, keys are like labels (e.g., “name”), and values are the data (e.g., “Alice”). When trying to call data and values from a dictionary, use “.get()
” to avoid errors in case a key that you have called doesn’t exist. A great tip to remember is that dictionaries are able to store lists or other dictionaries as values.
Classes and Objects
Classes are templates for creating objects. They let you group data and actions along with definitions and functions together and let you make them all relatedrelated to a singular name so it’sit’s easier to assign definitions and functions.
Example:
class Dog:
def __init__(self, name):
self.name = name
def bark(self):
return f"{self.name} says Woof!"
my_dog = Dog("Buddy")
print(my_dog.bark()) # Output: Buddy says Woof!
Tips:
Use classes to group related data and actions. Object-Oriented Programming (OOP) is especially useful for organizing larger projects because it allows you to model real-world concepts, reuse code efficiently, and keep your codebase clean. For instance, classes can represent different parts of a game, like a player, an enemy, or the game board, each with its own data and behaviors. Classes are used to define what objects can and cannot do. Use methods to add actions to your objects.
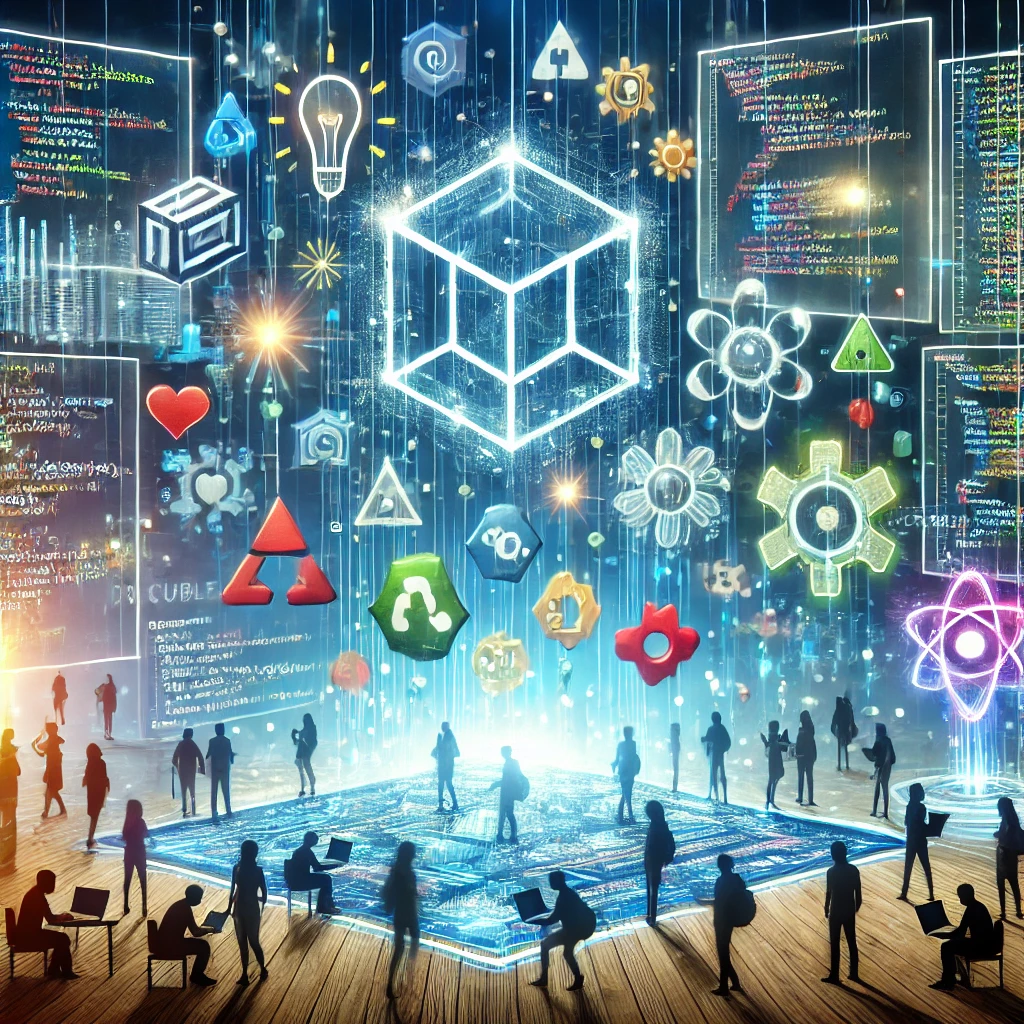
Conclusion
These coding basics – variables, data types, operators, lists, dictionaries, loops, functions, conditionals, and classes – are the tools you’ll use on a very regular basis to write programs. Each of these coding tactics plays an important role in solving problems in code and the real world and building software. Practice each one thoroughly to build your skills and confidence. Explore tutorials, experiment with small projects, and don’t be afraid to make mistakes. The more you code, the easier it gets. Happy coding!
Footnotes:
All images that haven’t been referenced were generated by AI